Celebrating 50,000 Stars: The Evolution of React
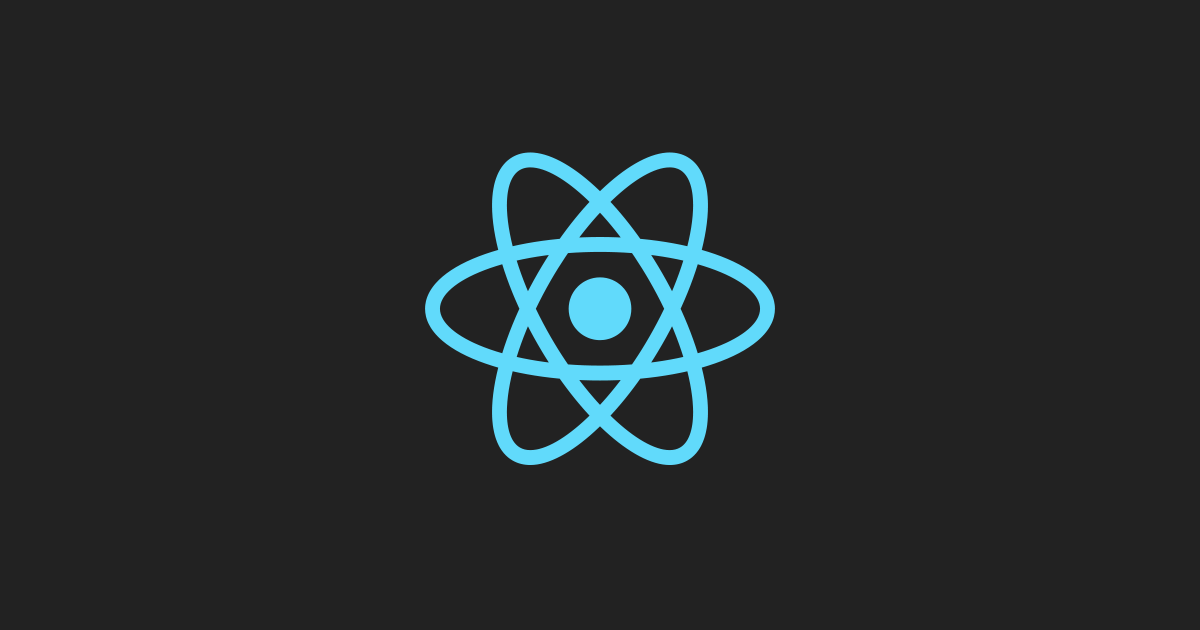
This blog site has been archived. For the latest updates, please visit While we have invested significant time in elucidating the concepts behind React and the challenges it addresses, we havent delved deeply into its evolution prior to becoming open-source. This milestone is a perfect opportunity to revisit the foundational moments and fun anecdotes from React's genesis, starting from the very first commits.
The story of React begins within our advertising organization, where we were developing sophisticated client-side web applications using an internal MVC framework known as BoltJS. To illustrate, consider the following sample of Bolt code:
var CarView = require ( 'javelin/core' ) . createClass ( { name : 'CarView' , extend : require ( 'container' ) . Container , properties : { wheels : 4 , } , declare : function ( ) { return { childViews : [ { content : 'I have ' } , { ref : 'wheelView' } , { content : ' wheels' } ] } ; } , setWheels : function ( wheels ) { this . findRef ( 'wheelView' ) . setContent ( wheels ) ; } , getWheels : function ( ) { return this . findRef ( 'wheelView' ) . getContent ( ) ; } , } ) ; var car = new CarView ( ) ; car . setWheels ( 3 ) ; car . placeIn ( document . body ) ;
Bolt introduced several APIs and features that would eventually find their way into React, such as render, createClass, and refs. The concept of refs was particularly noteworthy, as it allowed for the creation of references to nodes for imperative use. This capability was beneficial for legacy interoperability and the incremental adoption of new paradigms, although React would later evolve to embrace a more functional approach. Nonetheless, the use of refs proved invaluable in certain contexts where functional constraints were too limiting.
As our applications became increasingly intricate, our Bolt codebases began to exhibit significant complexity. Recognizing the limitations of this framework, Jordan Walke initiated a side project dubbed FaxJS, aimed at resolving many of the challenges presented by Bolt, but through a drastically different methodology. This endeavor led to the birth of several fundamental concepts that are now core to React, including props, state management, efficient tree re-evaluation to diff the user interface, server-side rendering, and the foundational idea of components.
TestProject . PersonDisplayer = { structure : function ( ) { return Div ( { classSet : { personDisplayerContainer : true } , titleDiv : Div ( { classSet : { personNameTitle : true } , content : this . props . name } ) , nestedAgeDiv : Div ( { content : 'Interests: ' + this . props . interests } ) } ) ; } } ;
As Jordan continued to refine his FaxJS experiment, he became increasingly convinced that adopting functional APIswhich discourage mutationwould provide a more effective and scalable solution for building user interfaces. In March 2012, he integrated his library into Facebooks codebase, rebranding it as FBolt. This new name signified an extension of Bolt, where components were crafted in a functional programming style. However, whether FBolt was a nod to FaxJS or simply a new designation remains an open question, as Jordan never clarified!
The seamless interoperability between FBolt and Bolt enabled us to incrementally replace components with the new functional component APIs, allowing us to cautiously explore this innovative paradigm without a full commitment. We started with components that were inherently more suited for expression in a functional style, gradually pushing the limits of functional expression within our code.
However, recognizing that FBolt might not be an ideal standalone name, Jordan Walke and Tom Occhino collaboratively proposed a new moniker: React. After Tom shared a diff of the renaming changes, Jordan added a remark that further justified the choice:
I might add for the sake of discussion, that many systems advertise some kind of reactivity, but they usually require that you set up some kind of point-to-point listeners and wont work on structured data. This API reacts to any state or property changes, and works with data of any form (as deeply structured as the graph itself) so I think the name is fitting.
At this time, Tom was also actively committing to the first version of GraphiQL, a project that has since been open-sourced.
Since around 2010, Facebook has utilized an extension of PHP called XHP, allowing engineers to construct UIs using XML literals directly within their PHP code. Initially introduced to mitigate XSS vulnerabilities, it turned out to be an excellent framework for structuring applications with custom components.
final class : a : post extends : x : element { attribute : a ; protected function render ( ) : XHPRoot { $anchor = < a > { $ this - > getChildren ( ) } a > ; $form = ( < form method = " post " action = { $ this - > : href } target = { $ this - > : target } class = " postLink " > { $anchor } form > ) ; $ this - > transferAllAttributes ( $anchor ) ; return $form ; } }
Before Jordans innovations made their way into Facebooks codebase, Adam Hupp had already devised an XHP-like concept for JavaScript, constructed in Haskell. This approach enabled developers to write structured code directly within JavaScript files:
function : example : hello ( attrib , children ) { return ( < div class = " special " > < h1 > Hello, World! h1 > { children } div > ) ; }
This code would compile into ES3-compatible JavaScript as follows:
function jsx_example_hello ( attrib , children ) { return ( S . create ( "div" , { "class" : "special" } , [ S . create ( "h1" , { } , [ "Hello, World!" ] ) , children ] ) ) ; }
In this prototype, the S.create function would instantly create and return a DOM node. Most discussions surrounding this prototype focused on comparing the performance of innerHTML with that of direct DOM node creation. At that time, it was not particularly favorable to push developers toward creating DOM nodes directly, as this method exhibited poor performanceespecially in browsers like Firefox and Internet Explorer. Facebooks then-CTO, Bret Taylor, weighed in on the conversation, advocating for the use of innerHTML over document.createElement:
If you are not convinced about innerHTML, here is a small microbenchmark. It is about the same in Chrome. innerHTML is about 30% faster in the latest version of Firefox (much more in previous versions), and about 90% faster in IE8.
Although this work was ultimately abandoned, it was revived following Reacts integration into the codebase. Jordan shifted focus away from previous performance discussions by introducing the concept of a Virtual DOM, although this term had not yet been formally defined.
As the project advanced, several alterations occurred in the component APIs and naming conventions during React's first year of development. For instance, the project underwent multiple name changes: it was initially known as declare, then structure, and ultimately settled on render. Similarly, component creation transitioned from componentize to createComponent and finally to createClass.
Just before the project was ready for open-sourcing, a crucial refactoring initiative took place, led by Lee Byron, in collaboration with Jordan Walke, Tom Occhino, and Sebastian Markbge. This effort aimed to overhaul Reacts lifecycle API, resulting in a well-structured API that continues to be a hallmark of React today.
In 2012, the acquisition of Instagram by Facebook served as a significant turning point for React. Pete Hunt, who was involved with Facebook photos and videos at that time, joined Instagrams newly formed web team. His ambition was to build their website entirely utilizing React, contrasting sharply with the incremental adoption strategy previously employed at Facebook.
To facilitate this vision, React needed to be decoupled from Facebooks existing infrastructure, which Instagram did not utilize. This project acted as a catalyst, prompting the necessary work to ready React for open-source release. During this process, Pete also discovered and endorsed a project called webpack, and he implemented the renderToString primitive essential for server-side rendering.
As preparations for the open-source launch were underway, designer Maykel Loomans crafted a mockup of what the Instagram website could look like, which ultimately set the visual identity for React, influencing its logo and the signature electric blue color.
In its formative days, React thrived on valuable feedback, ideas, and contributions from early adopters within the company. While it may appear as if React achieved overnight success, its story exemplifies how innovative concepts often require numerous iterations, refinements, and course corrections over extended periods before they can realize their true potential. The approach React has taken toward building user interfacesgrounded in functional programming principleshas dramatically transformed our development practices in just a few short years. Its important to acknowledge that React owes much of its success to the vibrant open-source community that has flourished around it!